API Documentation Classic and REST Versions
Online Forms Intro
This section contains descriptions for the web services to create, lookup, and delete L2P Widget requests.
The linked2pay (L2P) Web Services section below provides the developer a means to create Data Widget records in linked2pay. The records give you the ability to associate payment data from your existing system with linked2pay so that your customers can quickly and easily make payments to you through linked2pay simply by providing their identifier assigned by you (such as customer account numbers, invoice numbers, etc.) as a method for identifying themselves and the payments that they owe. linked2pay lets your customers make secure payments, provides them automated email receipts for their payments and provides the appropriate responses back to you so that you can update your accounting or reporting system.
As your customers make payments, the following web services also allow you to retrieve payment details so that you can update your systems as well as collect your customers email addresses for marketing and use with linked2pay Connect Web Service to email requests for payment directly to your customers.
For more information about linked2pay's API please email us at: supportteam@linked2pay.com
Getting Started
linked2pay makes getting started as easy as possible. Please use the following instructions:
- Review this document in its entirety.
Review the WSDL as well as our .NET sample application provided with your documentation.
To download your sample application click here
- Once you are ready to move to production, please register your client(s) by clicking “Register” on www.linked2pay.com and completing our simple 5 step registration process.
- Begin using the L2PWebService and start accepting payments!
If you would like to try out the JSON-oriented REST Web Service Features, click here to try, no account registration needed.
Overall
For an outline of step by step instructions on using the L2PWidget Webservice Sample Application review the PDF file provided along with your sample application.
To download your sample application click here
Methods
Name | Description |
---|---|
authenticate | Authenticate client by using his/her login name and KEY/FINGERPRINT. Clients must register with linked2pay to receive their credentials before using the L2P web services. Registration can be accessed at www.linked2pay.com |
createWidgetHeader |
Create widget header. Each widget request includes up to two (2) lookup fields. These are fields that the client can use to identify each request. For example, a client can specify “Account” or “Zip code”. To define what lookup fields are used for a particular L2P request and display the meaningful labels on the online form, the client has to create widget header upfront and reference this header in the L2P request. |
deleteWidgetRequest | This method gives you the ability to delete L2P widget request |
findWidgetRequest | This method lets you find a specific L2P widget request by providing a request ID. |
lookupWidget | This lists all widgets created by a particular client |
lookupWidgetHeader | This lists all headers created by a particular client (see createWidgetHeader method). |
lookupWidgetRequest | This lists all L2P requests created by a particular client which have specific particular values in the lookup fields |
submitWidgetAttachment | Used to upload a document (ex. PDF Invoice) as an attachment for a particular L2P widget request |
submitWidgetRequest | Create L2P widget request |
Description
Authenticate client by using the KEY Authentication or FINGERPRINT Authentication method. Registration can be accessed at www.linked2pay.com.
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
Returns
Name | Type | Description |
---|---|---|
emailLength | int | Maximum length of email that can be sent to the client |
status | short | Authentication result: 0 - success, negative value represents error code |
userId | long | System internal user id |
Remarks
Authentication is part of every L2P web service call and typically, there is no need to call this method directly.
Status represents an error code if its value is negative.
<?php define('L2P_SERVICE_PATH','http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT',L2P_SERVICE_PATH.'services/L2PWidgetWebService'); define('L2P_WSDL',L2P_SERVICE_PATH.'wsdl/L2PWidgetWebService.wsdl'); // SOAP client $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object $auth = new StdClass(); $auth->login = "mikel1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; // These parameters are specific to what function is being called $params = new StdClass(); $params->auth = $auth; $result = $client->authenticate($params); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Authentication Request</title> </head> <body> <div> <p>Response:</p> <div> <?php print $result->authenticateReturn; ?> </div> </div> </body> </html>
Description
Create widget header.
Each widget request includes up to two (2) lookup fields. These are fields that the client can use to identify each request. For example, a client can specify “Account” or “Zip code”.
To define what lookup fields are used for a particular L2P request and display the meaningful labels on the online form, the client has to create widget header upfront and reference this header in the L2P request.
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
label1 | string | Input | Lookup field label 1 |
label2 | string | Input | Lookup field label 2 |
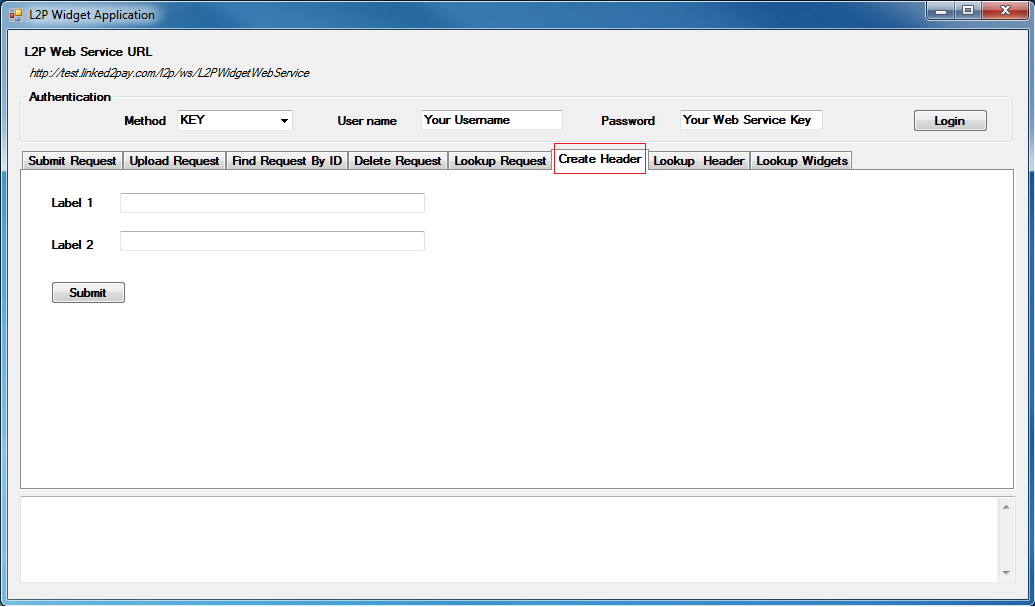
Returns
Numeric value (int) that represents return code (0 is successful execution, otherwise the number represents an error code) - see Attachment
Example
Below is the PHP code example:
<?php define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PWidgetWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PWidgetWebService.wsdl'); // SOAP client $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object $auth = new StdClass(); $auth->login = "mikel1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; // These parameters are specific to what function is being called $params = new StdClass(); $params->auth = $auth; $params->dataId = isset($_GET['dataId']) ? $_GET['dataId'] : 60; $result = $client->deleteWidgetRequest($params); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Delete Widget Request</title> </head> <body> <div> <p>Response:</p> <div> <?php print $result->deleteWidgetRequestReturn; ?> </div> </div> </body> </html>
Description
This method gives you the ability to delete L2P widget requests.
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
dataId | long | Input | ID of the L2P widget request to be deleted through deleteWidgetRequest method. |
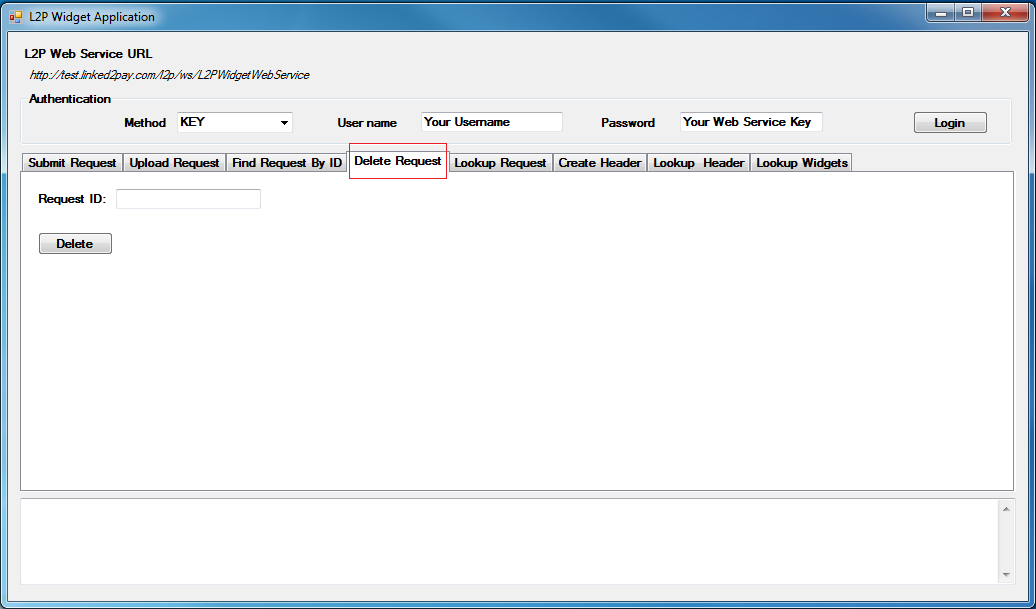
Returns
Numeric value (int) that represents return code (0 is successful execution, otherwise the number represents an error code) - see Attachment
Remarks
The L2P request ID is returned by submitWidgetRequest method or can be obtained through findWidgetRequest and/or lookupWidgetRequest methods.
Example
Below is the PHP code example:
<?php define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PWidgetWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PWidgetWebService.wsdl'); // SOAP client $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object $auth = new StdClass(); $auth->login = "client1"; $auth->password = "client1"; // These parameters are specific to what function is being called $params = new StdClass(); $params->auth = $auth; $params->label1 = isset($_GET['label1']) ? $_GET['label1'] : 'Richard Account'; $params->label2 = isset($_GET['label2']) ? $_GET['label2'] : 'Richard Zip'; $result = $client->createWidgetHeader($params); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Create Widget Header</title> </head> <body> <div> <p>Response:</p> <div> <?php print $result->createWidgetHeaderReturn; ?> </div> </div> </body> </html>
Description
This method lets you find a specific L2P widget request by providing a request ID.
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
dataId | long | Input | ID of the L2P widget request which information is retrieved through findWidgetRequest method. |
Returns
Data object having the structure defined by the following table.
Name | Type | Description |
---|---|---|
status | int | Request status: negative value represents error code |
headerId | long | Header ID points to an existing L2P widget header |
widgetId | long | Widget ID points to an existing L2P widget |
lookup1 | string | Lookup field is part of L2PWidgetRequest object and serves to further identify the particular L2P request(s). |
lookup2 | string | Lookup field is part of L2PWidgetRequest object and serves to further identify the particular L2P request(s). |
description | string | L2P request description (for example “Monthly Invoice”) |
startDate | string | Date on which L2P request becomes active |
endDate | string | Date on which L2P request expires |
pastDueDate | string | Date on which L2P payment is due |
amount | double |
Amount requested to be paid. Numeric value with 2 decimal points |
pastDueAmount | double |
Amount requested to be paid after due date. Numeric value with 2 decimal points |
createdDate | string | Date when L2P request has been submitted. |
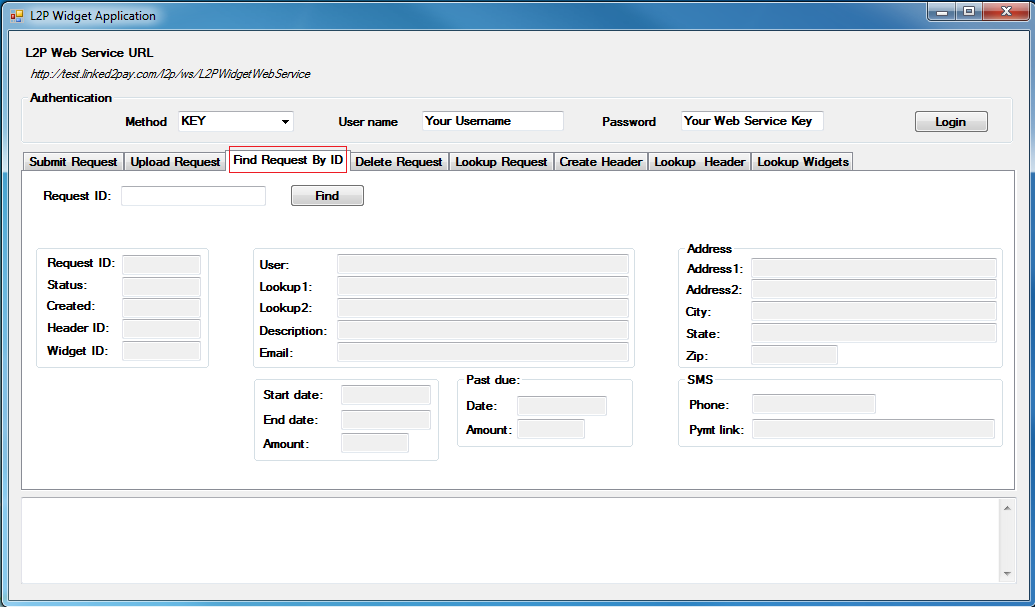
Remarks
L2PWidgetRequest object contains field status - this field reflects request status or error code if value is negative.
Example
Below is the PHP code example:
<?php define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PWidgetWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PWidgetWebService.wsdl'); // SOAP client $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object $auth = new StdClass(); $auth->login = "mikel1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; // These parameters are specific to what function is being called $params = new StdClass(); $params->auth = $auth; $params->dataId = isset($_GET['dataId']) ? $_GET['dataId'] : 73; $result = $client->findWidgetRequest($params); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Find Widget Request</title> </head> <body> <div> <p>Response:</p> <div> <?php foreach ($result->findWidgetRequestReturn as $k => $v) print $k.' -- <b>'.$v.'</b><br />'."\n"; ?> </div> </div> </body> </html>
Description
This lists all widgets created by a particular client.
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
Returns
Name | Type | Description |
---|---|---|
L2PWidget | L2PWidget | Data object that includes header attributes |
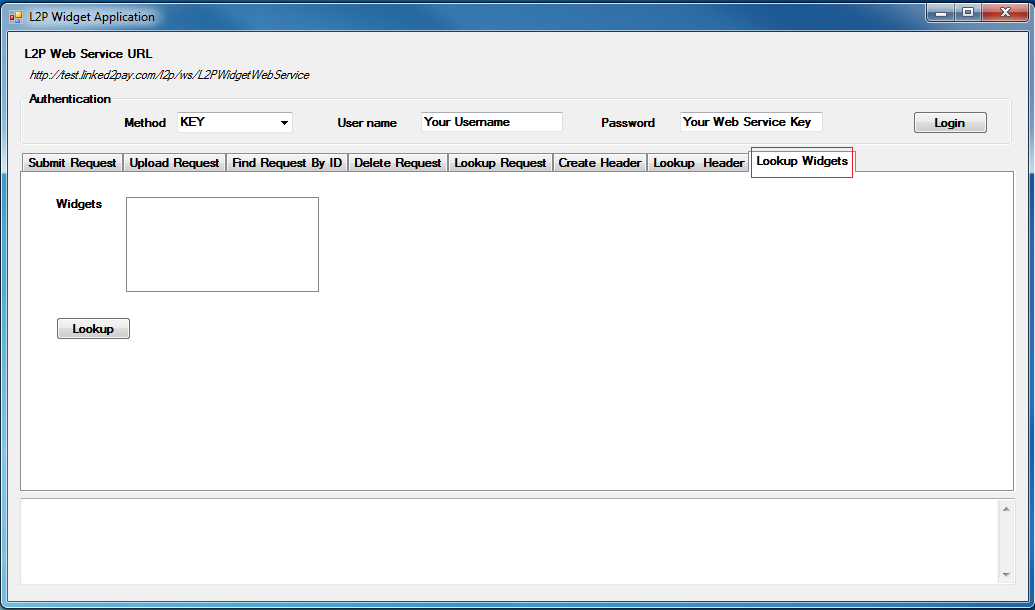
Remarks
The widget can be generated by the client through the Widget Builder section of the L2P portal. The code generated can be integrated into the clients’ website.
If an error occurs, this method still returns an array that has one 'empty' L2PWidget object with status set to a specified error code.
Example
Below is the PHP code example:
<?php define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PWidgetWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PWidgetWebService.wsdl'); // SOAP client $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object $auth = new StdClass(); $auth->login = "mikel1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; // these parameters are specific to what function is being called $params = new StdClass(); $params->auth = $auth; $result = $client->lookupWidget($params); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Lookup Widget</title> </head> <body> <div> <p>Response:</p> <div> <?php if(is_array($result->lookupWidgetReturn->L2PWidget)) { foreach ($result->lookupWidgetReturn->L2PWidget as $obj) { print_results($obj); print '<hr />'."\n"; } } else { print_results($result->lookupWidgetReturn->L2PWidget); } ?> </div> </div> </body> </html>
Description
This lists all headers created by a particular client (see createWidgetHeader method).
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
Returns
Name | Type | Description |
---|---|---|
L2PHeader | L2PHeader | Data object that includes header attributes. |
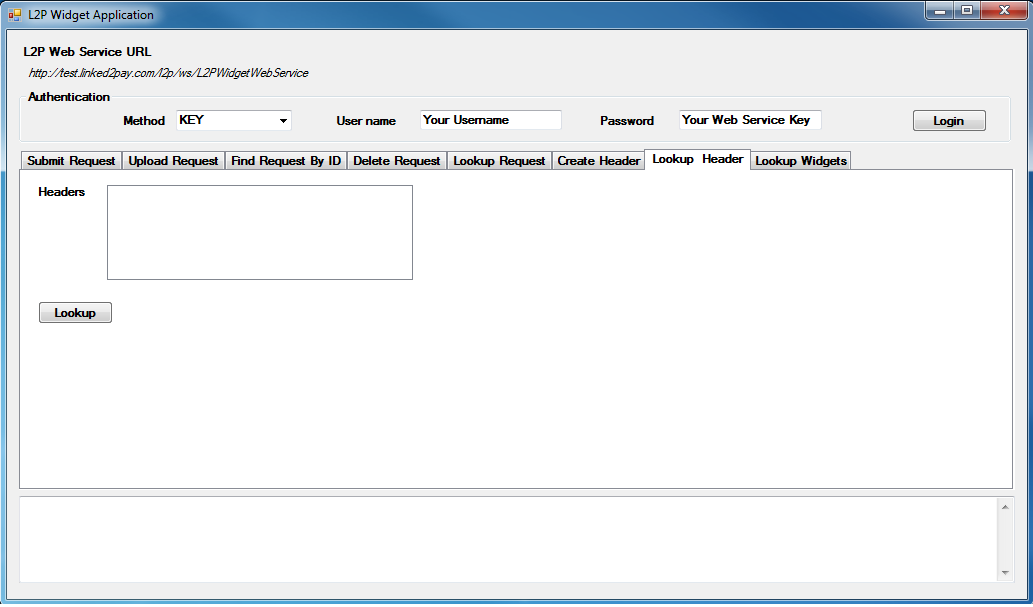
Remarks
L2PHeader object contains field status - this field reflects header status or error code if value is negative.
If error occurs this method still returns an array that has one 'empty' L2PHeader object with status set to an error code.
Example
Below is the PHP code example:
<?php define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PWidgetWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PWidgetWebService.wsdl'); // SOAP client $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object $auth = new StdClass(); $auth->login = "mikel1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; // these parameters are specific to what function is being called $params = new StdClass(); $params->auth = $auth; $result = $client->lookupWidgetHeader($params); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Lookup Header</title> </head> <body> <div> <p>Response:</p> <div> <?php if(is_array($result->lookupHeaderReturn->L2PHeader)) { foreach ($result->lookupHeaderReturn->L2PHeader as $obj) { print_results($obj); print '<hr />'."\n"; } } else { print_results($result->lookupHeaderReturn->L2PHeader); } ?> </div> </div> </body> </html>
Description
This lists all L2P requests created by a particular client which have specific particular values in the lookup fields
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
lookup1 | string | Input | Lookup value 1 |
lookup2 | string | Input | Lookup value 2 |
Returns
Name | Type | Description |
---|---|---|
L2PWidgetRequest | L2PWidgetRequest | Data object that includes widget request attributes. |
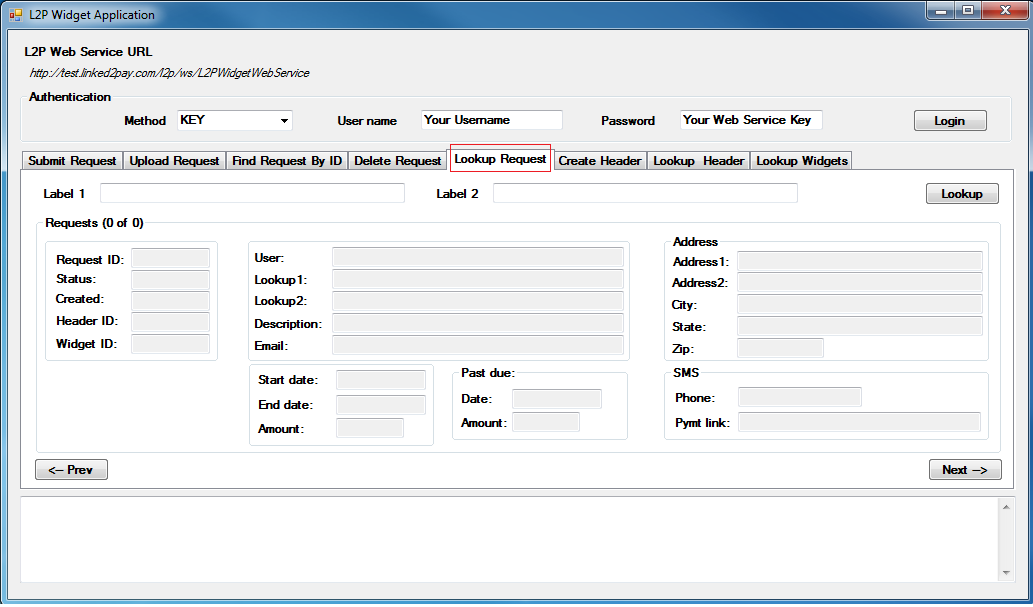
Remarks
L2PWidgetRequest object contains field status - this field reflects request status or error code if value is negative. If error occurs this method still returns an array that has one 'empty' L2PWidgetRequest object with status set to an error code.
Example
Below is the PHP code example:
<?php define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PWidgetWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PWidgetWebService.wsdl'); // SOAP client $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object $auth = new StdClass(); $auth->login = "mikel1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; // these parameters are specific to what function is being called $params = new StdClass(); $params->auth = $auth; $params->lookup1 = isset($_GET['lookup1']) ? $_GET['lookup2'] : '0000000001'; $params->lookup2 = isset($_GET['lookup1']) ? $_GET['lookup2'] : '66666'; $result = $client->lookupWidgetRequest($params); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Lookup Widget Request</title> </head> <body> <div> <p>Response:</p> <div> <?php if(is_array($result->lookupWidgetRequestReturn->L2PWidgetRequest)) { foreach ($result->lookupWidgetRequestReturn->L2PWidgetRequest as $obj) { print_results($obj); print '<hr />'."\n"; } } else { print_results($result->lookupWidgetRequestReturn->L2PWidgetRequest); } ?> </div> </div> </body> </html>
Description
Used to upload a document (ex. PDF Invoice) as an attachment for a particular L2P widget request
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
attachment | L2PWidgetAttachment | Input | Data object that contains attachment attributes |
attachmentData | base64Binary | Input | Base 64 encoded binary data (type byte) |
Returns
Numeric value (int) that represents return code (0 is successful execution, otherwise the number represents an error code) - see Attachment
Example
Below is the PHP code example:
<?php if(isset($_POST['submit'])) { define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PWidgetWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PWidgetWebService.wsdl'); // SOAP client $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object $auth = new StdClass(); $auth->login = "client1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; $attachment = new StdClass(); $attachment->fileName = $_FILES['userUpload']['name']; $attachment->filePassword = 'stephen'; $attachment->passwordHint = 'name'; $attachment->dataId = isset($_POST['dataId']) ? $_POST['dataId'] : 73; $attachmentData = file_get_contents($_FILES['userUpload']['tmp_name']); $encodedData = base64_encode($attachmentData); // These parameters are specific to what function is being called $params = new StdClass(); $params->auth = $auth; $params->attachment = $attachment; $params->attachmentData = $encodedData; $result = $client->submitWidgetAttachment($params); } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Submit Widget Request Attachment</title> </head> <body> <div style="background: white;"> <?php if(isset($_POST['submit'])) { ?> <p>Response:</p> <div> <?php print $result->submitWidgetAttachmentReturn; ?> </div> <div> </div> <?php } else { ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post" enctype="multipart/form-data"> <div> <input type="file" name="userUpload" /> </div> <div> <input type="submit" name="submit" value="Upload File" /> </div> </form> <?php } ?> </div> </body> </html>
Description
Create L2P widget request.
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
request | L2PWidgetRequest | Input | Data object that includes widget request attributes |
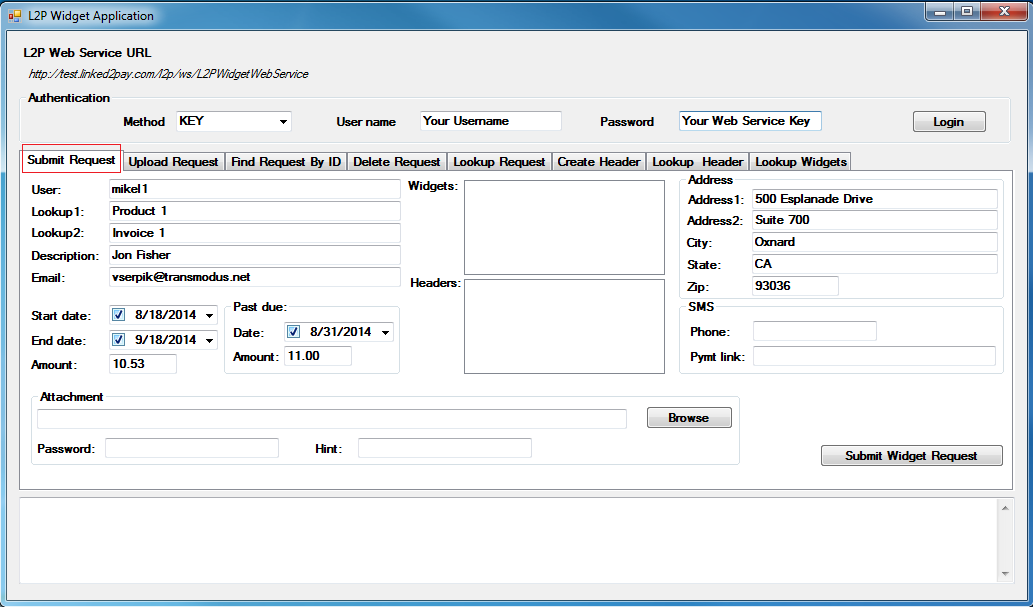
Returns
Numeric value (int) that represents return code (0 is successful execution, otherwise the number represents an error code) - see Attachment
Example
Below is the PHP code example:
<?php define('L2P_SERVICE_PATH', 'http://test.transmodus.net/jcx/soa/test/'); define('L2P_ENDPOINT', L2P_SERVICE_PATH.'services/L2PWidgetWebService'); define('L2P_WSDL', L2P_SERVICE_PATH.'wsdl/L2PWidgetWebService.wsdl'); // SOAP client $client = new soapclient(L2P_WSDL, array('location' => L2P_ENDPOINT, 'trace' => 1, 'exceptions' => 0)); // Authentication object $auth = new StdClass(); $auth->login = "client1"; $auth->AuthenticationMethod = "KEY"; $auth->password = "0123456789ABCDEF"; $l2pWidgetRequest = new StdClass(); $l2pWidgetRequest->status = 0; $l2pWidgetRequest->headerId = 71; $l2pWidgetRequest->widgetId = 0; $l2pWidgetRequest->lookup1 = '8058860687'; $l2pWidgetRequest->lookup2 = '11111'; $l2pWidgetRequest->description = 'Richard Submission'; $l2pWidgetRequest->startDate = '06/08/2012'; $l2pWidgetRequest->endDate = ''; $l2pWidgetRequest->pastDueDate = ''; $l2pWidgetRequest->amount = 500.00; $l2pWidgetRequest->pastDueAmount = 0.00; // these parameters are specific to what function is being called $params = new StdClass(); $params->auth = $auth; $params->request = $l2pWidgetRequest; $result = $client->submitWidgetRequest($params); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <title>L2P Submit Widget Request</title> </head> <body> <div> <p>Response:</p> <div> <?php print $result->submitWidgetRequestReturn; ?> </div> </div> </body> </html>
Complex Types
Name | Description |
---|---|
L2PAuthenticationData | Data object that contains authentication information based on user's choice of authentication method. |
L2PAuthenticationResponse |
Object returned by authenticate method. It contains status, user ID and email maximum length. |
L2PHeader |
Object returned by lookupHeader method. It represents information about L2P header including header Id, status and labels for two lookup fields - see headerId, status, label1, label2 |
L2PWidget |
Object returned by lookupWidget method. It contains data about L2P widget that client placed on his portal HTML page. Data include widget ID, name and status - see widgetId, name, status. |
L2PWidgetAttachment | Object contains attachment attributes and passed as input parameter of submitWidgetAttachment method. |
L2PWidgetRequest | Object passed as input parameter of submitWidgetRequest method or returned by findWidgetRequest and lookupWidgetRequest methods. |
fingerprintBlock | A bundle of information, containing user's sequence number, timestamp and a 16-character generated fingerprint |
Description
Object returned by authenticate method.
It contains status, user ID and email maximum length.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
emailLength | int | 1..1 | Maximum length of email that can be sent to the client |
login | string | 1..1 | L2P client login name up to 20 characters |
status | short | 1..1 | Authentication result: 0 - success, negative value represents error code |
userId | long | 1..1 | System internal user id |
fingerprint | fingerprintBlock | 0..1 | A bundle of information, containing user's sequence number, timestamp and a 16-character generated fingerprint |
Description
Object returned lookupHeader method.
It represents information about L2P header including header Id, status and labels for two lookup fields - see headerId, status, label1, label2.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
status | int | 1..1 | Negative error code or 0 if call is successful. |
headerId | long | 1..1 | Header ID returned as part of L2PHeader object by lookupHeader method |
label1 | string | 1..1 | Lookup field label returned as part of L2PHeader object by lookupHeader method |
label2 | string | 1..1 | Lookup field label returned as part of L2PHeader object by lookupHeader method |
Description
Object returned by lookupWidget method.
It contains data about L2P widget that client placed on his portal HTML page. Data includes widget ID, name and status - see widgetId, name, status.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
status | int | 1..1 | Widget status: negative value represents error code |
widgetId | long | 1..1 | Widget ID returned as part of L2PWidget object by lookupWidget method |
name | string | 1..1 | Name of the client L2P widget |
Description
Object contains attachment attributes and passed as input parameter of submitWidgetAttachment method.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
fileName | string | 1..1 |
Name of the attachment file. System uses file name extension to detect attachment type (pdf,txt,etc.) This field is required. |
filePassword | string | 1..1 |
Password up to 20 characters. If specified will be requested on the linked2pay payment page to open/view the attachment. This field is optional. |
passwordHint | string | 1..1 |
Password hint up to 50 characters. If specified could be requested on the L2P portal to open/view the attachment. This field is optional. |
dataId | long | 1..1 |
ID of a widget request submitted attachment will be associated with. This field is required. |
Description
Object passed as input parameter of submitWidgetRequest method or returned by findWidgetRequest and lookupWidgetRequest methods.
Content Model
Contains elements as defined in the following table:
Component | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
status | int | 1..1 | Request status: negative value represents error code |
headerId | long | 1..1 | Header ID points to an existing L2P widget header. |
widgetId | long | 1..1 | Widget ID points to an existing L2P widget |
lookup1 | string | 1..1 | Lookup field is part of L2PWidgetRequest object and serves to further identify the particular L2P request(s). |
lookup1 | string | 1..1 | Lookup field is part of L2PWidgetRequest object and serves to further identify the particular L2P request(s). |
lookup2 | string | 1..1 | Lookup field is part of L2PWidgetRequest object and serves to further identify the particular L2P request(s). |
description | string | 1..1 | L2P request description (for example “Monthly Invoice”) |
startDate | string | 1..1 | Date on which L2P request becomes active |
endDate | string | 1..1 | Date on which L2P request expires |
pastDueDate | string | 1..1 | Date on which L2P payment is due. |
amount | double | 1..1 |
Amount requested to be paid. Numeric value (type double) with 2 decimal points |
pastDueAmount | double | 1..1 |
Amount requested to be paid after due date. Numeric value (type double) with 2 decimal points |
createdDate | string | 1..1 | Date when L2P request has been submitted. |
Other Elements
Web Service Possible Error Codes
This is a list of possible error codes you may see when using the Online Forms, Email Payments, or Reporting Web Services.
ERROR CODES
Description
Negative error code or 0 if call is successful.
See full list of possible error codes list below:
Note: This list of error codes applies to the L2P Widget Webservice, L2P Payment Webservice, and the L2P Reporting Webservice
Possible error codes:
L2P_OK = 0
L2P_DB_EXCP = -1
L2P_USER_NOT_EXIST = -2
L2P_USER_ACCESS_DENIED = -3
L2P_REQUEST_NOT_EXIST = -4
L2P_REQUEST_ACCESS_DENIED = -5
L2P_NO_REQUESTS_FOUND = -6
L2P_CONTACT_NOT_EXIST = -7
L2P_EMAIL_FAILED = -8
L2P_CONTACT_OPT_OUT = -9
L2P_EMAIL_TOO_LONG = -10
L2P_PAYMENT_URL_MISSING = -11
L2P_SYSTEM_ERROR = -12
L2P_HEADER_NOT_EXIST = -13
L2P_NO_ATTACHMENT = -14
L2P_NO_HEADERS_FOUND = -15
L2P_NO_WIDGETS_FOUND = -16
L2P_COMPANY_NAME_TOO_LONG = -17
L2P_LAST_NAME_NOT_PRESENT = -18
L2P_LAST_NAME_TOO_LONG = -19
L2P_FIRST_NAME_NOT_PRESENT = -20
L2P_FIRST_NAME_TOO_LONG = -21
L2P_EMAIL_INVALID = -22
L2P_EMAIL_NOT_PRESENT = -23
L2P_AMOUNT_NOT_PRESENT = -24
L2P_CC_INVALID = -25
L2P_CC_TOO_LONG = -26
L2P_BCC_INVALID = -27
L2P_BCC_TOO_LONG = -28
L2P_PHONE_TOO_LONG = -29
L2P_TEMPLATE_NAME_TOO_LONG = -30
L2P_EMAIL_SUBJECT_TOO_LONG = -31
L2P_EMAIL_BODY_TOO_LONG = -32
L2P_ADDRESS1_NOT_PRESENT = -33
L2P_ADDRESS1_TOO_LONG = -34
L2P_ADDRESS2_TOO_LONG = -35
L2P_CITY_NOT_PRESENT = -36
L2P_CITY_TOO_LONG = -37
L2P_STATE_NOT_PRESENT = -38
L2P_STATE_TOO_LONG = -39
L2P_ZIP_NOT_PRESENT = -40
L2P_ZIP_TOO_LONG = -41
L2P_ZIP_INVALID = -42
L2P_GL_BANK_TOO_LONG = -43
L2P_GL_EXPENSE_TOO_LONG = -44
L2P_GL_DESC_TOO_LONG = -45
L2P_INVOICE_TOO_LONG = -46
L2P_EMAIL_MESSAGE_TOO_LONG = -47
L2P_TEMPLATE_INVALID = -48
L2P_EMAIL_SUBJECT_NOT_PRESENT = -49
L2P_EMAIL_BODY_NOT_PRESENT = -50
L2P_WIDGET_REQUEST_INVALID_HEADER = -101
L2P_WIDGET_REQUEST_INVALID_LOOKUP1 = -102
L2P_WIDGET_REQUEST_INVALID_LOOKUP2 = -103
L2P_WIDGET_REQUEST_INVALID_DESCRIPTION = -104
L2P_WIDGET_REQUEST_INVALID_START_DATE = -105
L2P_WIDGET_REQUEST_INVALID_END_DATE = -106
L2P_WIDGET_REQUEST_INVALID_PAST_DUE_DATE = -107
L2P_WIDGET_REQUEST_INVALID_AMOUNT = -108
L2P_WIDGET_REQUEST_INVALID_PASTDUE_AMOUNT = -109
L2P_WIDGET_REQUEST_INVALID_PHONE_NUMBER = -110
L2P_WIDGET_REQUEST_INVALID_PYMT_LINK = -111
L2P_WIDGET_REQUEST_INVALID_PHONE_NUMBER_OR_PYMT_LINK = -112
L2P_WIDGET_REQUEST_INVALID_EMAIL = -113
L2P_WIDGET_REQUEST_INVALID_OPTOUT_EMAIL = -114
L2P_WIDGET_REQUEST_INVALID_STREET1 = -115
L2P_WIDGET_REQUEST_INVALID_STREET2 = -116
L2P_WIDGET_REQUEST_INVALID_CITY = -117
L2P_WIDGET_REQUEST_INVALID_STATE = -118
L2P_WIDGET_REQUEST_INVALID_ZIP = -119
L2P_WIDGET_REQUEST_INVALID_ADDRESS = -120
L2P_WIDGET_REQUEST_INVALID_USER = -121
L2P_WIDGET_REQUEST_INVALID_USER_ACCOCIATION = -122
L2P_WIDGET_REQUEST_INVALID_WIDGET = -123
L2P_WIDGET_REQUEST_INVALID_MOBILE_NOLOOKUP_NOMAP_TEMPLATE = -124
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_LOOKUP1_REQUIRED = -125
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_ADDRESS1_REQUIRED = -126
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_CITY_REQUIRED = -127
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_STATE_REQUIRED = -128
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_ZIP_REQUIRED = -129
L2P_ATTACHMENT_INVALID_PASSWORD = -140
L2P_ATTACHMENT_INVALID_HINT = -141
L2P_ATTACHMENT_INVALID_FILE_NAME = -142
L2P_WIDGET_DATA_ID_NOT_PRESENT = -143
L2P_REQUEST_ID_NOT_PRESENT = -144
Call our Online Forms WebService to add a single invoice manually or an infinite number of invoices through an upload process. Other than the addition of invoices you have the ability to use our Online Forms WebService to update or remove any invoice attachment
Resource Quick Links