API Documentation Classic and REST Versions
Reporting WebService Intro
The L2P Reporting WebService is a method that allows you to retrieve request details pertaining to your linked2pay requests. You can drill down on your requests based on different criteria such as Request ID, Submission Date, Amount, etc.
While Credit Card provides instant response of accepted or declined status, ACH transactions have a life cycle that make this tool essential for retrieving changes in transaction status
To get started follow these instructions:
- Review this documentation and in its entirety
- Download the L2P Reporting Webservice Sample Application by clicking here
-
Download the WSDL file and review it in its entirety
To obtain the corresponding WSDL for testing purposes click here
- Once you are ready to move to production, please register your client(s) by clicking "Register" on www.linked2pay.com and completing our simple 5 step registration process.
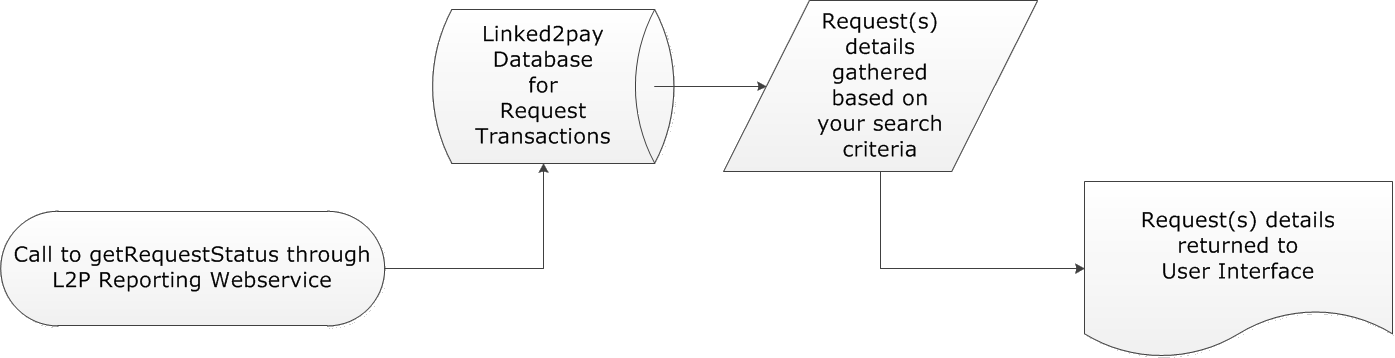
For more information about linked2pay's API please email us at: supportteam@linked2pay.com
If you would like to try out the JSON-oriented REST Web Service Features, click here to try, no account registration needed.
Overall
Authentication will take place before a method is called. Only authenticated users are able to execute these methods. Click here to learn about how authentication works.
This Webservice contains one method that allows you to retrieve information about your linked2pay payment requests based on the search parameters you set.
Methods
Name | Description |
---|---|
getRequestStatus | Get L2P request(s) information including all payments related to the request(s). |
The “getRequestStatus” method can be used to gather linked2pay request(s) details and all payment information for the request(s). You can search for request(s) details by Request ID, Status, Submission Date, Amount, etc.
Parameters
Name | Type | Direction | Description |
---|---|---|---|
auth | L2PAuthenticationData | Input | Data object that contains authentication information based on user's choice of authentication method. |
l2pRequestInquiry | L2PRequestInquiry | Input | Data objects that include inquiry criteria. |
Returns
Name | Type | Description |
---|---|---|
errorCode | int |
Negative error code or 0 if call is successful. See error codes list in the attachment. |
requestStatus | L2PRequestStatusList | Array of objects that contain L2P request(s) information. |
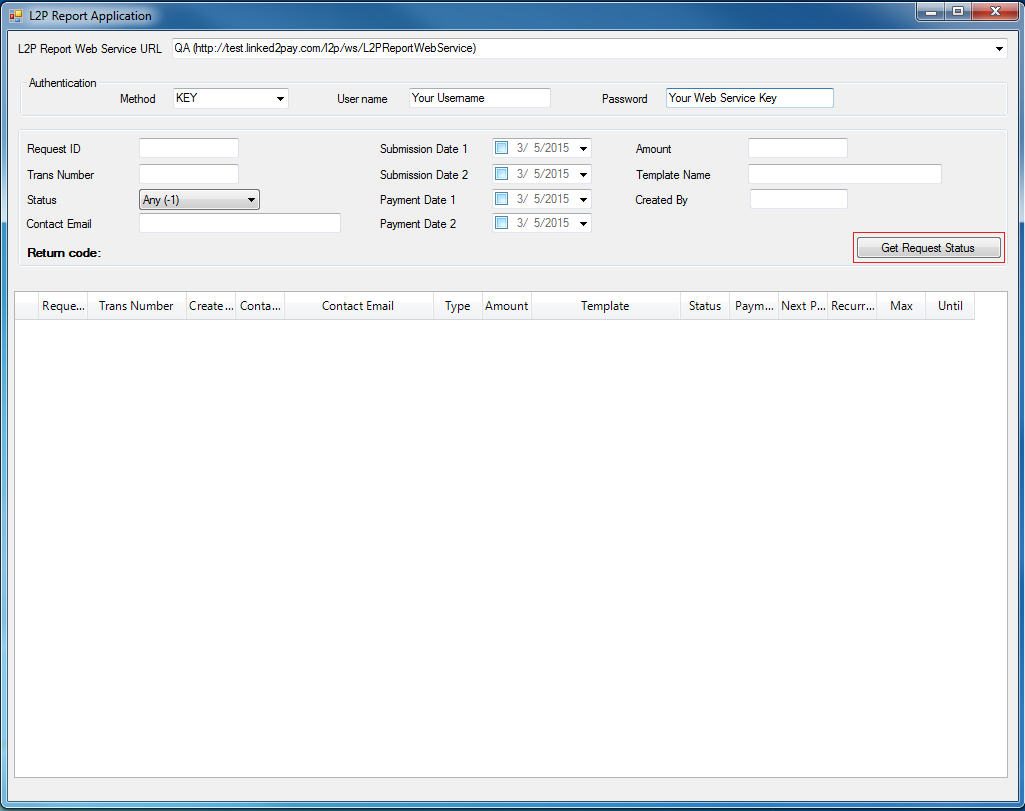
Below is the code example in C#
// Web Reference has been added as: // reference name: L2PReportWebService // URL: http://test.transmodus.net/jcx/soa/test/wsdl/L2PReportWebService.wsdl // More on adding service references see: // http://msdn.microsoft.com/en-us/library/bb628649.aspx // http://msdn.microsoft.com/en-us/library/bb628652.aspx using System; using L2PPaymentSampleApplication.L2PPaymentWebService; namespace L2PReportSampleApplication { class Program { static void Main(string[] args) { L2PReportWebServiceService l2pReportWebService = new L2PReportWebServiceService(); l2pReportWebService .Url = http://test.transmodus.net/jcx/soa/test/services/L2PReportWebService; L2PReportWebServiceService.L2PAuthenticationData auth = new L2PReportWebServiceService.L2PAuthenticationData(); auth.login = "mikel1"; // auth.AuthenticationMethod = "KEY"; // password = "0123456789ABCDEF"; L2PReportWebService.fingerprintBlock fingerprintBlock = new L2PReportWebService.fingerprintBlock(); FingerprintGenerator fingerprintGenerator = new FingerprintGenerator(); fingerprintBlock.sequence = fingerprintGenerator.GetSuquenceNumber(); fingerprintBlock.fingerprint = fingerprintGenerator.GenerateFingerPrint( (int)fingerprintBlock.sequence, fingerprintBlock.timesatmp.ToString(), "0123456789ABCDEF", auth.login); auth.fingerprint = fingerprintBlock; L2PReportWebServiceService.L2PRequestInquiry l2pRequestInquiry = new L2PReportWebServiceService.L2PRequestInquiry(); l2pRequestInquiry.requestId = 12345; l2pRequestInquiry.status = -1; l2pRequestInquiry.amount = 0; l2pRequestInquiry.submissionDate1 = ""; l2pRequestInquiry.submissionDate2 = ""; l2pRequestInquiry.paymentDate1 = ""; l2pRequestInquiry.paymentDate2 = ""; l2pRequestInquiry.templateName =""; l2pRequestInquiry.createdBy = 0; 2PReportWebService.L2PResponse l2pResponse = l2pReportWebService.getRequestStatus(auth, l2pRequestInquiry); Console.WriteLine("Error code: " + l2pDebitResponse.errorCode); L2PReportWebService.L2PRequestStatus[] l2pRequestStatusArr = l2pResponse.requestStatus; if (l2pRequestStatusArr != null) { for (int i = 0; i < l2pRequestStatusArr.Length; i++) { L2PReportWebService.L2PRequestStatus l2pRequestStatus = l2pRequestStatusArr[i]; Console.WriteLine(l2pRequestStatus.requestId); Console.WriteLine(l2pRequestStatus.createdBy); Console.WriteLine(l2pRequestStatus.contactId); Console.WriteLine(l2pRequestStatus.contactEmail); Console.WriteLine(l2pRequestStatus.paymentType); Console.WriteLine(l2pRequestStatus.amount); Console.WriteLine(l2pRequestStatus.templateName); Console.WriteLine(l2pRequestStatus.status); Console.WriteLine(l2pRequestStatus.paymentCnt); Console.WriteLine(l2pRequestStatus.nextPaymtDate, Console.WriteLine(l2pRequestStatus.recurringFrequency); Console.WriteLine(l2pRequestStatus.maxPayments); Console.WriteLine(l2pRequestStatus.untilDate); GridViewTemplate template = radGridView.MasterGridViewTemplate.ChildGridViewTemplates[0]; L2PReportWebService.L2PPaymentStatus[] l2pPaymentStatusArr = l2pRequestStatus.l2pPaymentStatus; if (l2pPaymentStatusArr != null) { for (int j = 0; j < l2pPaymentStatusArr.Length; j++) { L2PReportWebService.L2PPaymentStatus l2pPaymentStatus = l2pPaymentStatusArr[j]; Console.WriteLine(l2pRequestStatus.requestId); Console.WriteLine(l2pPaymentStatus.paymtId); Console.WriteLine(l2pPaymentStatus.paidAmount); Console.WriteLine(l2pPaymentStatus.paymentMethod); Console.WriteLine(l2pPaymentStatus.submissionDate); Console.WriteLine(l2pPaymentStatus.creditCardType); Console.WriteLine(l2pPaymentStatus.creditCardNumber); Console.WriteLine(l2pPaymentStatus.cardHolder); Console.WriteLine(l2pPaymentStatus.expirationMonth); Console.WriteLine(l2pPaymentStatus.expirationYear); Console.WriteLine(l2pPaymentStatus.achRequestId); Console.WriteLine(l2pPaymentStatus.routeNumber); Console.WriteLine(l2pPaymentStatus.accountNumber); Console.WriteLine(l2pPaymentStatus.accountType); Console.WriteLine(l2pPaymentStatus.depositType); Console.WriteLine(l2pPaymentStatus.achSubmissionDate); Console.WriteLine(l2pPaymentStatus.achEffectiveDate); } } } } } } }
Complex Types
Name | Description |
---|---|
L2PAuthenticationData | Data object that contains authentication information based on user's choice of authentication method. |
L2PCustomVariable | Data object that contains template custom variables. |
L2PCustomVariableList | JAX_WS holder object. |
L2PPaymentStatus | Data object that contains payment information. |
L2PPaymentStatusList | JAX_WS holder object. |
L2PRequestInquiry | Data object that defines parameters for your query. |
L2PRequestStatus | Data object that contains request information. |
L2PRequestStatusList | JAX_WS holder object. |
L2PResponse | Response object with information on submitted request. |
Description
Data object that contains template custom variable(s).
Content Model
Contains elements as defined in the following table:
Name | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
variableName | string> | 1..1 | Name of the custom variable. |
variableValue | string | 1..1 | Value of the custom variable. |
Description
JAX_WS holder object.
Content Model
Contains elements as defined in the following table:
Name | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
L2PCustomVariable | L2PCustomVariable | 0..* | Users list of custom variables and values. |
Remarks
DotNet Webservice consumers will retrieve L2PPaymentStatus objects as array by passing this folder.
Description
Data object that contains payment information.
Content Model
Contains elements as defined in the following table:
Name | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
paymtId | long | 1..1 | System internal payment ID that can be used to communicate with L2P customer service. |
paidAmount | double | 1..1 | Amount Paid on a request. |
paymentMethod | string | 1..1 |
Payment method: A - ACH C - credit card |
paymentSeq | short | 1..1 | Not implemented. |
submissionDate | string | 1..1 | Date payment has been submitted. |
creditCardType | string | 1..1 | Type of credit card used for payment. |
creditCardNumber | string | 1..1 | Four last digits of the credit card. |
cardHolder | string | 1..1 | Name of Credit Card holder. |
expirationMonth | string | 1..1 | Expiration month of the credit card being used. |
expirationYear | string | 1..1 | Expiration year of the credit card being used. |
achRequestId | long | 1..1 | System internal ACH request ID that can be used to communicate with L2P customer service. |
routeNumber | string | 1..1 | Route Number of the bank account used for payment. |
accountNumber | string | 1..1 | Last four numbers of the bank account used for payment. |
accountType | string | 1..1 |
Type of bank account used for payment: P - personal B - business |
depositType | string | 1..1 |
Deposit type: C - checking S - savings |
achSubmissionDate | string | 1..1 | Actual date the ACH request has been submitted for processing. |
achEffectiveDate | string | 1..1 | Effective Date when funds will be available in your (merchant's) bank account. |
Description
JAX_WS holder object.
Content Model
Contains elements as defined in the following table:
Name | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
L2PPaymentStatus | L2PPaymentStatus | 0..* | Status of payments submitted in response to your request. |
Remarks
DotNet Webservice consumers will retrieve L2PCustomVariable objects as array by passing this folder.
Description
Data object that defines the parameters of your query.
Content Model
Contains elements as defined in the following table:
Name | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
requestId | long | 1..1 | System Internal unique Request Identification number assigned to each of your Webservice requests. |
transNumber | string | 1..1 | Unique Transaction Number that is assigned to each of your requests and can be used to communicate with linked2pay customer service. |
status | short | 1..1 |
Request status. |
contactEmail | string | 1..1 | Your customers email address. |
amount | double | 1..1 | Amount of your request. |
submissionDate1 | string | 1..1 | Date the requests have been submitted. |
submissionDate2 | string | 1..1 |
Date the requests have been submitted. If this parameter is specified then all requests submitted between submissionDate1 and submissionDate2 are retrieved. |
paymentDate1 | string | 1..1 | Date the payments have been submitted |
paymentDate2 | string | 1..1 |
Date the payments have been submitted. If this parameter is specified then all requests that have payments submitted between paymentDate1 and paymentDate2 are retrieved. |
templateName | string | 1..1 | Name of the L2P template used to create payment request(s). |
createdBy | long | 1..1 | System internal User Identification Number of the client User that created the payment request(s). |
Remarks
These search parameters can be used individually or in unison.
Description
Data object that contains request information.
Content Model
Contains elements as defined in the following table:
Name | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
requestId | long | 1..1 | System Internal unique Request Identification number assigned to each of your Webservice requests. |
transNumber | string | 1..1 | Unique Transaction Number that is assigned to each of your requests and can be used to communicate with linked2pay customer service. |
createdBy | long | 1..1 | System internal User Identification Number of the client User that created the payment request(s). |
contactId | long | 1..1 | System internal Identification number of the customer this request has been addressed to. |
contactEmail | string | 1..1 | Your customers email address. |
paymentType | string | 1..1 |
Type of transaction against your account: D - debit C - credit |
amount | double | 1..1 | Amount of your Request. |
templateName | string | 1..1 | Name of the L2P template used to create payment request(s). |
status | short | 1..1 |
Request status. |
paymentCnt | short | 1..1 | Number of payment related to this request. |
nextPaymtDate | string | 1..1 | Date when the next payment is scheduled to be submitted for processing. |
recurringFrequency | string | 1..1 |
Recurring frequency. Empty for non-recurring requests. M - monthly, W - weekly, Q - quarterly, Y - yearly |
untilDate | string | 1..1 | The date that marks the end of a recurring payment cycle. |
lookup1 | string | 1..1 | Lookup value 1. |
lookup2 | string | 1..1 | Lookup value 2. |
l2pPaymentStatus | L2PPaymentStatusList | 1..1 | Array of objects that contain payment details. |
l2pCustomVariables | L2PCustomVariableList | 1..1 | Array of objects that contain template custom variables and values. |
Description
JAX_WS holder object.
Content Model
Contains elements as defined in the following table:
Name | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
L2PRequestStatus | L2PRequestStatus | 0..* | Detailed information of your linked2pay request. |
Referenced By
Name | Type |
---|---|
requestStatus [type L2PResponse] | Element |
Description
Response object with information on submitted request.
Content Model
Contains elements as defined in the following table:
Name | Type | Occurs | Description |
---|---|---|---|
SEQUENCE | 1..1 | ||
errorCode | int | 1..1 |
Negative error code or 0 if call is successful. See error codes list in the L2P request status section. |
requestStatus | L2PRequestStatusList | 1..1 | Array of objects that contain L2P Request Status details. |
Referenced By
Name | Type |
---|---|
requestStatus [type L2PResponse] | Element |
L2P REQUEST STATUS
Description
Array of objects that contain L2P request(s) information.
Remarks
Instance | Status | Description |
---|---|---|
0 | Pending |
Request has been made (request remains in this status only if email failed). |
1 | Active | Email has been sent out to the customer with a link to the payment page. |
2 | Clicked | Customer clicked the link in the email he received and got the payment page. |
3 | Submitted ACH | ACH transaction is ready to be processed. |
4 | Paid ACH | ACH transaction has been processed. |
5 | Returned | ACH transaction has come back as a return. |
6 | Expired | Email has been sent, but customer did not click the link within the allowed number of days to pay so payment link is no longer active. |
7 | Sent to Paper | Client marked request (that has not been paid yet) to be taken to paper. |
8 | Opt-out | Customer stated that he does not want to receive email requests and pay electronically |
9 | Failed | Request processing failed. |
10 | Submitted CC | Credit card transaction has been submitted. |
11 | Paid CC | Credit card transaction has been processed. |
12 | Sold Out | First ‘N’ customers have been processed (campaign style). |
13 | Transcribe ACH | Request has been submitted and check has been uploaded through the mobile phone application. |
14 | Submit ACH | ACH request submitted through mobile phone is ready to be processed (similar to 3). |
15 | Refunded CC | Credit card transaction has been reversed. |
16 | Check Mailed | Credit payment request has been made but contact has not provided his ACH account through the payment page. |
17 | Paid By Check | Paper check has been sent to the Contact |
18 | Declined CC | Credit Card payment declined by processor. |
19 | Dispute | Your contact has decided to dispute all or part of your requested amount. Further action is required for you to collect payment. See invoice information for details. |
ERROR CODES
Description
Negative error code or 0 if call is successful.
See full list of possible error codes list below:
Note: This list of error codes applies to the L2P Widget Webservice, L2P Payment Webservice, and the L2P Reporting Webservice
Possible error codes:
L2P_OK = 0
L2P_DB_EXCP = -1
L2P_USER_NOT_EXIST = -2
L2P_USER_ACCESS_DENIED = -3
L2P_REQUEST_NOT_EXIST = -4
L2P_REQUEST_ACCESS_DENIED = -5
L2P_NO_REQUESTS_FOUND = -6
L2P_CONTACT_NOT_EXIST = -7
L2P_EMAIL_FAILED = -8
L2P_CONTACT_OPT_OUT = -9
L2P_EMAIL_TOO_LONG = -10
L2P_PAYMENT_URL_MISSING = -11
L2P_SYSTEM_ERROR = -12
L2P_HEADER_NOT_EXIST = -13
L2P_NO_ATTACHMENT = -14
L2P_NO_HEADERS_FOUND = -15
L2P_NO_WIDGETS_FOUND = -16
L2P_COMPANY_NAME_TOO_LONG = -17
L2P_LAST_NAME_NOT_PRESENT = -18
L2P_LAST_NAME_TOO_LONG = -19
L2P_FIRST_NAME_NOT_PRESENT = -20
L2P_FIRST_NAME_TOO_LONG = -21
L2P_EMAIL_INVALID = -22
L2P_EMAIL_NOT_PRESENT = -23
L2P_AMOUNT_NOT_PRESENT = -24
L2P_CC_INVALID = -25
L2P_CC_TOO_LONG = -26
L2P_BCC_INVALID = -27
L2P_BCC_TOO_LONG = -28
L2P_PHONE_TOO_LONG = -29
L2P_TEMPLATE_NAME_TOO_LONG = -30
L2P_EMAIL_SUBJECT_TOO_LONG = -31
L2P_EMAIL_BODY_TOO_LONG = -32
L2P_ADDRESS1_NOT_PRESENT = -33
L2P_ADDRESS1_TOO_LONG = -34
L2P_ADDRESS2_TOO_LONG = -35
L2P_CITY_NOT_PRESENT = -36
L2P_CITY_TOO_LONG = -37
L2P_STATE_NOT_PRESENT = -38
L2P_STATE_TOO_LONG = -39
L2P_ZIP_NOT_PRESENT = -40
L2P_ZIP_TOO_LONG = -41
L2P_ZIP_INVALID = -42
L2P_GL_BANK_TOO_LONG = -43
L2P_GL_EXPENSE_TOO_LONG = -44
L2P_GL_DESC_TOO_LONG = -45
L2P_INVOICE_TOO_LONG = -46
L2P_EMAIL_MESSAGE_TOO_LONG = -47
L2P_TEMPLATE_INVALID = -48
L2P_EMAIL_SUBJECT_NOT_PRESENT = -49
L2P_EMAIL_BODY_NOT_PRESENT = -50
L2P_CONTACT_ID_EMAIL_NOT_PRESENT = -52
L2P_WIDGET_REQUEST_INVALID_HEADER = -101
L2P_WIDGET_REQUEST_INVALID_LOOKUP1 = -102
L2P_WIDGET_REQUEST_INVALID_LOOKUP2 = -103
L2P_WIDGET_REQUEST_INVALID_DESCRIPTION = -104
L2P_WIDGET_REQUEST_INVALID_START_DATE = -105
L2P_WIDGET_REQUEST_INVALID_END_DATE = -106
L2P_WIDGET_REQUEST_INVALID_PAST_DUE_DATE = -107
L2P_WIDGET_REQUEST_INVALID_AMOUNT = -108
L2P_WIDGET_REQUEST_INVALID_PASTDUE_AMOUNT = -109
L2P_WIDGET_REQUEST_INVALID_PHONE_NUMBER = -110
L2P_WIDGET_REQUEST_INVALID_PYMT_LINK = -111
L2P_WIDGET_REQUEST_INVALID_PHONE_NUMBER_OR_PYMT_LINK = -112
L2P_WIDGET_REQUEST_INVALID_EMAIL = -113
L2P_WIDGET_REQUEST_INVALID_OPTOUT_EMAIL = -114
L2P_WIDGET_REQUEST_INVALID_STREET1 = -115
L2P_WIDGET_REQUEST_INVALID_STREET2 = -116
L2P_WIDGET_REQUEST_INVALID_CITY = -117
L2P_WIDGET_REQUEST_INVALID_STATE = -118
L2P_WIDGET_REQUEST_INVALID_ZIP = -119
L2P_WIDGET_REQUEST_INVALID_ADDRESS = -120
L2P_WIDGET_REQUEST_INVALID_USER = -121
L2P_WIDGET_REQUEST_INVALID_USER_ACCOCIATION = -122
L2P_WIDGET_REQUEST_INVALID_WIDGET = -123
L2P_WIDGET_REQUEST_INVALID_MOBILE_NOLOOKUP_NOMAP_TEMPLATE = -124
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_LOOKUP1_REQUIRED = -125
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_ADDRESS1_REQUIRED = -126
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_CITY_REQUIRED = -127
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_STATE_REQUIRED = -128
L2P_WIDGET_REQUEST_INVALID_MOBILE_TEMPLATE_ZIP_REQUIRED = -129
L2P_WIDGET_REQUEST_INVALID_EMAIL_REQUIRED = -132
L2P_ATTACHMENT_INVALID_PASSWORD = -140
L2P_ATTACHMENT_INVALID_HINT = -141
L2P_ATTACHMENT_INVALID_FILE_NAME = -142
L2P_WIDGET_DATA_ID_NOT_PRESENT = -143
L2P_REQUEST_ID_NOT_PRESENT = -144
The Report WebService allows you to drill down on your requests based on a series of search parameters that can be used individually or in unison. These search parameters include, but are not limited to, 'Request ID', 'Amount', 'Transaction Number', 'Contact Email', etc.
Resource Quick Links